Webhooks allow you to receive real-time updates when a specific event occurs, i.e. alert notification is sent for a monitored location. These events are HTTP POST requests being sent to an endpoint of your server. The payload body of such a request is in JSON format, similar to the Events API.
If you're new to webhooks, you can read this guide for more information.
Configuring a Webhook
- Sign in to the Tomorrow.io platform
- Select Development section
- Select the Webhooks tab
- You should see a screen similar to the one below which lists all the types of events you can subscribe to. At the moment, only "Alerts" are possible but keep an eye out for updates.
- For each webhook type you wish to subscribe to, enter a unique, publicly accessible URL in the standard format of
http(s)://(www.)domain.com/
and, if https, must have a valid SSL certificate - You are able to copy or rest a secret in order to authenticate the requests made to that URL
- Don't forget to click the "Add Webhook" button
- Make sure the status toggle is active
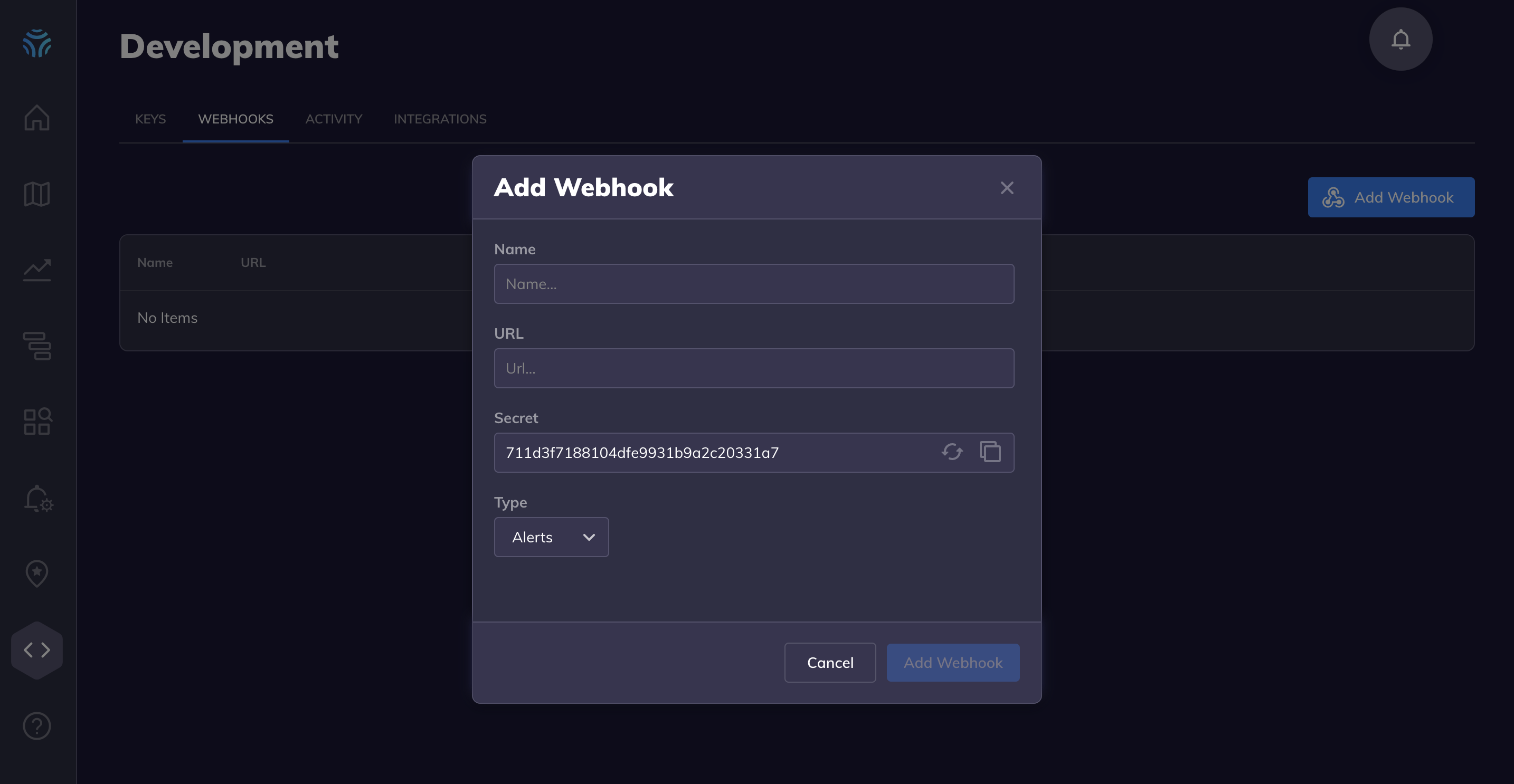
Response to Hooks
When you receive a webhook event, you should always return HTTP response in 2xx class, i.e. 200 OK in the shortest time possible. If your webhook fails with response code 4xx or 5xx or exceeds the maximum time, Tomorrow.io will try to re-deliver the webhook again up to two times with a few minutes in between each webhook. If all retries fail, the webhook call is discarded.
Check the Activity section in the Dashboard to debug any issues when setting up your webhooks.
Authenticate Hooks
When creating a webhook, Tomorrow.io will auto-generate a secret hash (which can be regenerated when needed). Every Tomorrow.io webhook request will have a secret sign, allowing you to validate that it was indeed from us. It is appended to a header name X-Signature
, built from two parts: the timestamp and the signature itself. This information can be used to verify that the webhook was sent from a known source.
You can also add an extra layer of security by whitelisting the IP address used by our worker node from which the webhook is sent: 35.231.166.26
In order to validate that webhook requests are signed with the secret, follow these steps:
- Extract the signature header and separate the
time
andsignature
- Calculate the signature according to the time in the header (as string) and the secret as the key, using the HMAC protocol with the SHA256 algorithm
- Compare the signature calculation to the provided signature header (byte-wise or on the hex string)
Here's an example code:
const crypto = require('crypto');
// secret that was appended to the webhook
const secret = '<your secret here>';
// signing the secret with the timestamp from the request
function sign(secret, timestamp) {
const hmac = crypto.createHmac('sha256', secret);
hmac.update(timestamp);
return hmac.digest('hex');
}
function webhook(req) {
// tomorrow.io signature header is "t={timestamp},sig={signature}"
const signatureHeader = req.headers['X-Signature'].split(',');
// extract timestamp
const timestamp = signatureHeader[0].split('=')[1];
// extract signature
const signature = signatureHeader[1].split('=')[1];
// getting the expected signature
const expectedSignature = sign(secret, timestamp);
if (signature !== expectedSignature) {
throw new Error();
}
}